Looking for a way to return more than one value from a Python function?
To return more than one value from a function in Python is impossible. What do you mean? What are you saying?
A python function always returns one value. A value is an object, and a function returns only one value. However, since the function returns an object, you decide what that object contains!
Let’s look at an example. We have a function my_func
which does one thing; it returns the integer value 1.
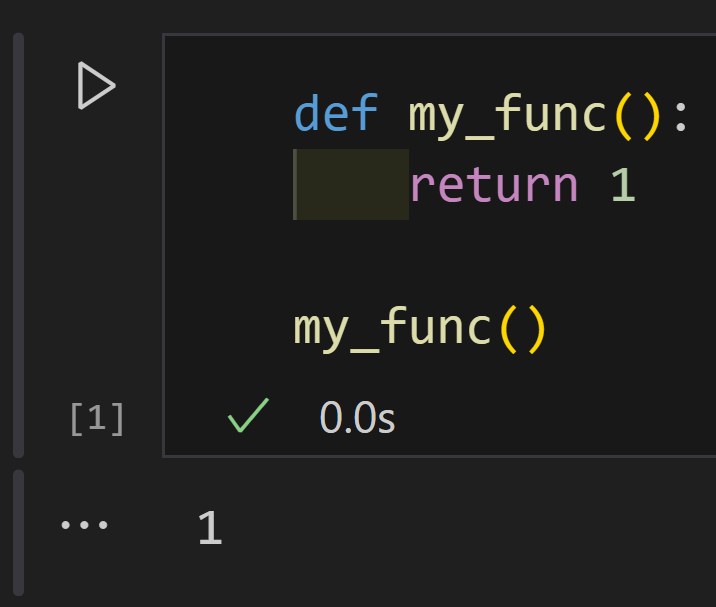
An integer is also an object, in this case a class named int
which we can check the type of by assigning the return value to a variable one
and passing it to type(one)
.
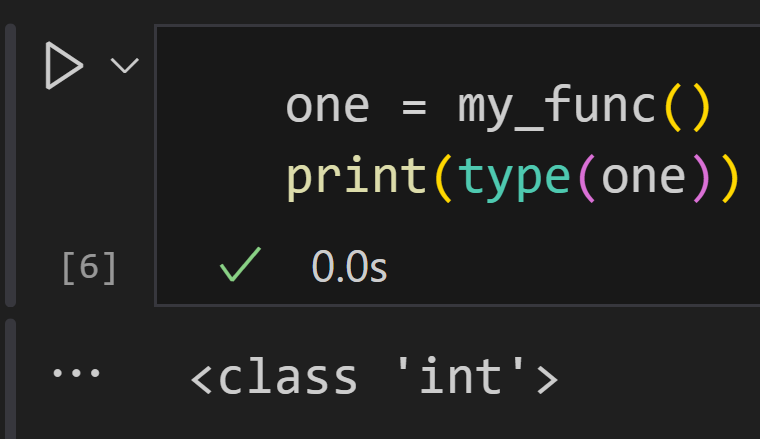
Okay, but how do I return multiple values in an object? We return an object which can hold multiple objects! Let’s define a function multiple_values
which returns the integers 1 and 2.
What do you think the return value will be? Returning two integers should give us the type int
right? Not quite, the object returned is a tuple
.
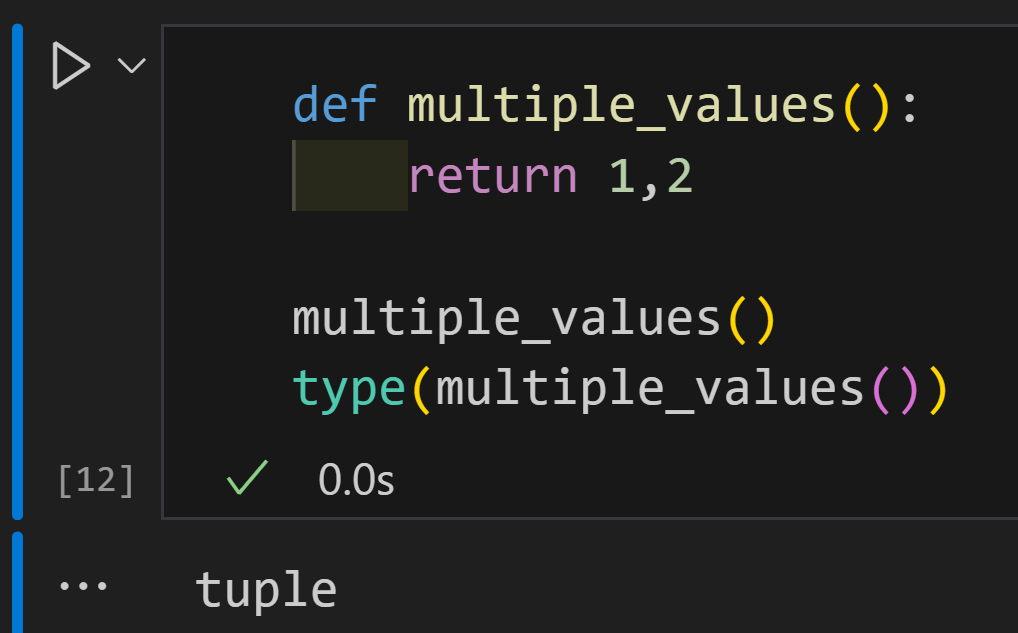
Python knows we want to return multiple values, because we actually return a tuple containing two integers from the function! Let’s dive into some Python syntax to explore this further.
Tuple syntax
Writing parentheses around your values declares a tuple. my_tuple = (1,2)
gives us a tuple of length two. But, you can also declare a tuple without the parentheses!
my_other_tuple = 1,2
also gives us a tuple. Returning the values 1,2 from a function means that we are creating a tuple and returning it from the function!
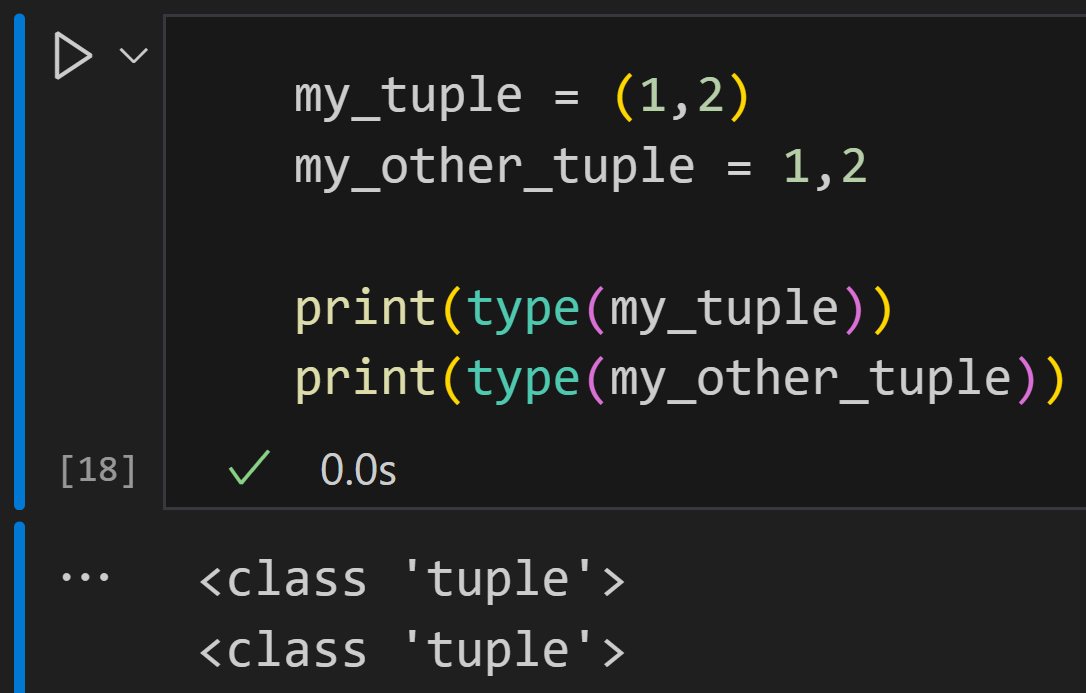
But why doesn’t it return a tuple when we return just one value? To return a tuple with only one value, we have to write a comma after the value.
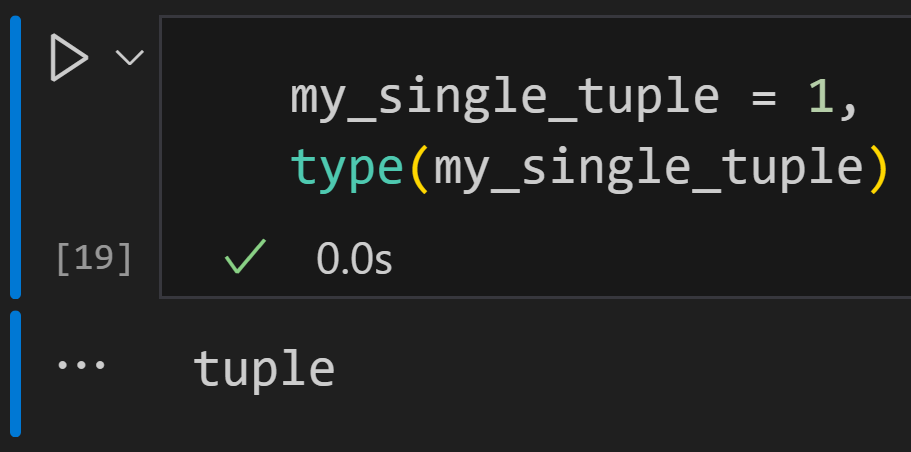
Next up, we will look at assigning values to our variables (unpacking values) from the tuple.
Unpacking values from a tuple
We looked at how we could return a tuple from a function, but how do we assign these values to variables? This is done by tuple unpacking.
Unpacking is done the same way as creating a tuple. We write the number of variables that we have to the left instead.
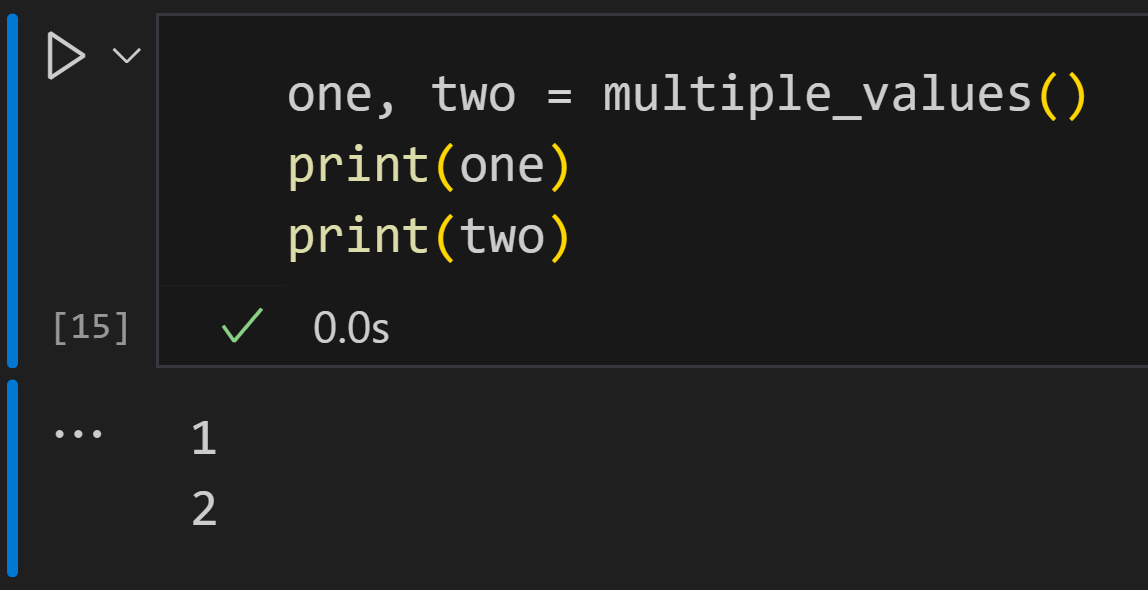
Wait a minute Conny! What happens when we do not know the number of items in a tuple? Good question, we use the asterisk * unpacking operator.
The asterisk * unpacking operator
In the example below, we create a tuple (1,2,3,4)
and assign it to the variable my_tuple
. We then unpack it to the variables one
, two
and *the_rest
. Note the asterisk on the variable the_rest
.
The asterisk tells Python to put all the remaining values in the tuple into a list. You can also unpack lists
and dictionaries
!
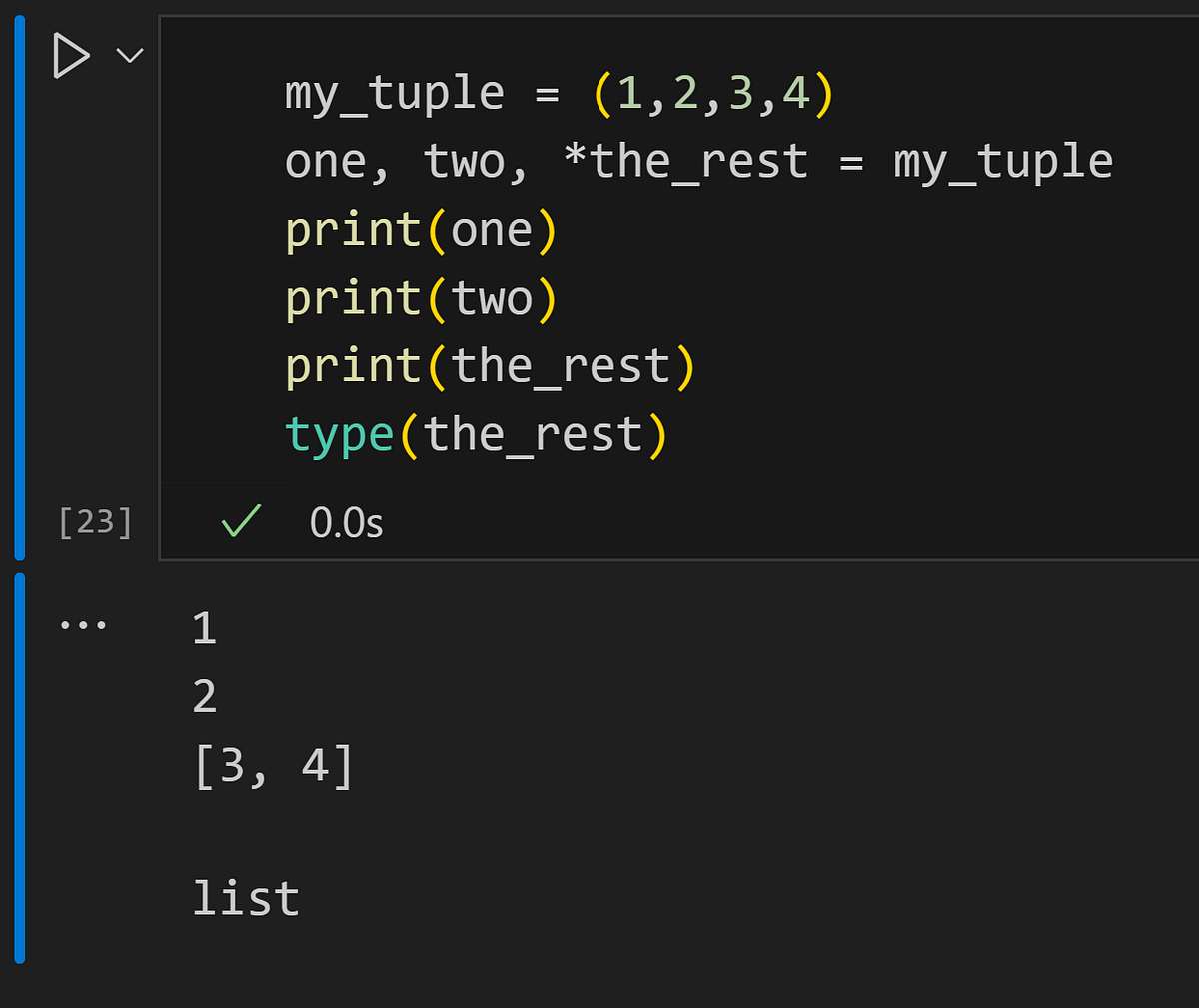
Returning a dictionary from your function
You can return a dictionary or any other object from your function. Here, we have a function called returning_a_dictionary
. We return a dictionary comprising four key-value pairs.
Assign the dictionary to a variable and you can use it straight away.
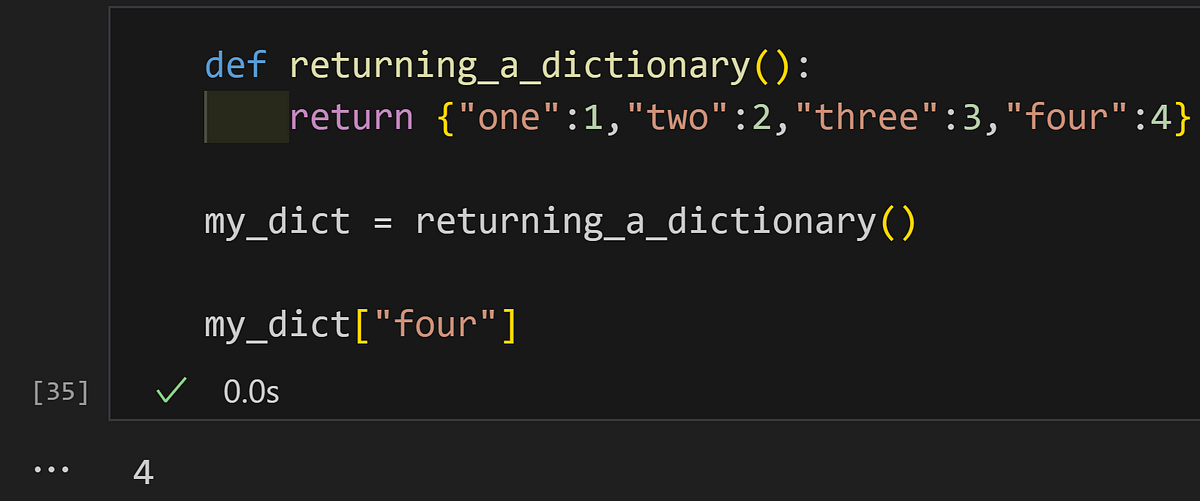
Source Code
Source code is available on my Github.
Conclusion
We looked at how Python returns tuples when specifying values separated by a comma. We learned how to unpack these tuples into variables and also how to handle unpacking of objects of unknown lengths.